Figure
Present visual content elegantly with Bramble UI's figure component. Elevate your web design with modern figure layouts and immersive visuals.
Usage
Using img
import {Figure} from '@/ui'
<Figure
caption='Beagle' // optional
className='max-w-sm' // set width
>
<img
src='/img/dogs/beagle.jpg'
title='Beagle'
alt='Beagle'
/>
</Figure>
Output
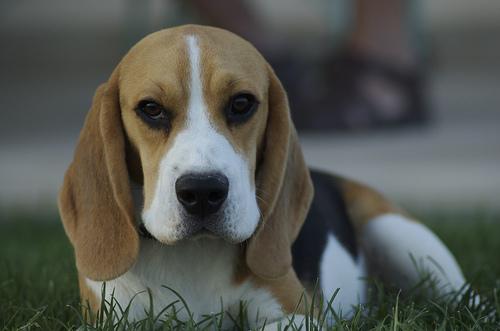
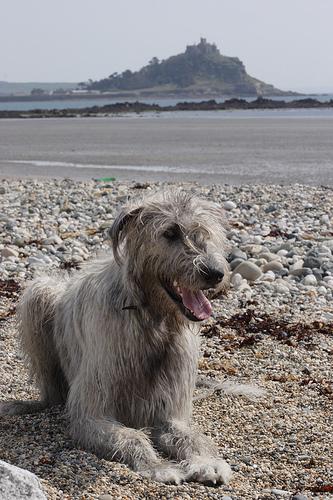
Using Nextjs Image
(known dimensions)
import Image from 'next/image'
import Figure from '@/components/figure'
<Figure
caption='Beagle'
className='max-w-sm'
>
<Image
width='500' // set width
height='320' // set height
src='/img/dogs/beagle.jpg'
title='Beagle'
alt='Beagle'
/>
</Figure>
Output
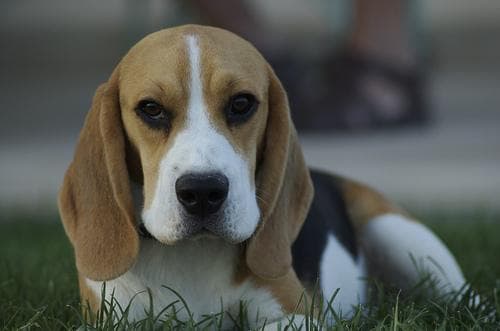
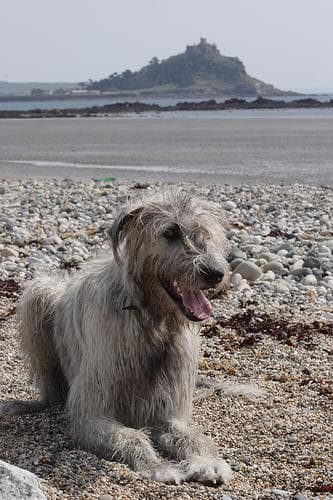
Remote image (size unknown)
<Image
fill // required
src='https://images.dog.ceo/breeds/beagle/n02088364_6866.jpg'
...
/>
<Image
fill
src='https://images.dog.ceo/breeds/wolfhound-irish/n02090721_847.jpg'
...
/>
Output - default aspect-[4/3]
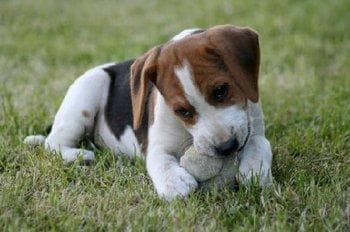
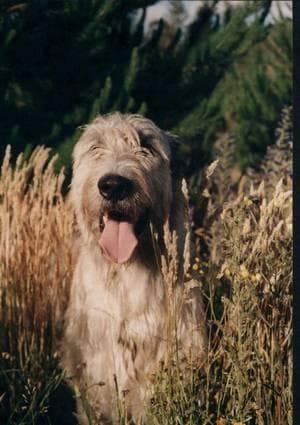
Output - portrait aspect-[3/4]
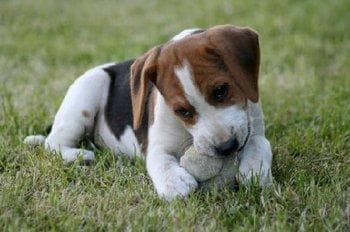
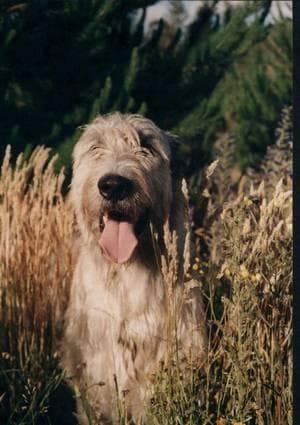
<Figure className='... portrait'
Square - aspect-[1/1]
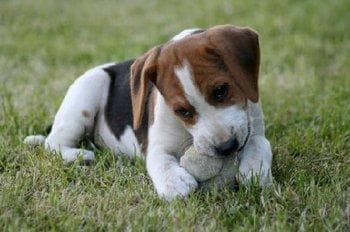
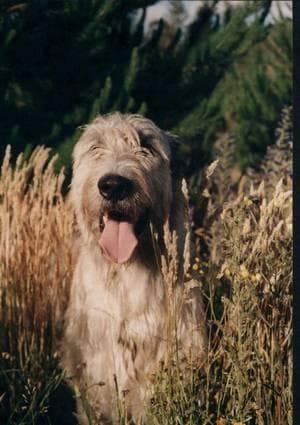
<Figure className='... square'
Video
<Figure
caption='Big Buck Bunny'
className='max-w-lg'
>
<Video
src='https://commondatastorage.googleapis.com/gtv-videos-bucket/sample/BigBuckBunny.mp4'
formats={['mp4', 'webm']}
/>
</Figure>
Output
Props
className?: string | undefined
children: React.ReactNode
caption?: string | undefined
CSS
.figure {
@apply w-full h-auto;
&:has(.video) {
@apply aspect-video relative;
}
&:has([data-nimg='fill']) {
@apply relative aspect-[4/3];
& img {
@apply w-full h-full object-cover;
}
&.portrait {
@apply aspect-[3/4];
}
&.square {
@apply aspect-square;
}
&:has(.figcaption) {
@apply mb-8
}
.figcaption {
@apply absolute -bottom-10 w-full;
}
}
.figcaption {
@apply p-2 text-center;
}
}